As I continue my journey in learning more about golang. I am venturing off to find frameworks that I can use to write better clis or apis. There is a popular framework called Cobra that helps golang developers generate cli applications that can scale. kubectl
, hugo
, and gh
are few of many that are leveraging Cobra.
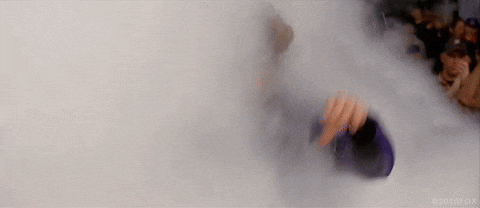
I do have a cookiecutter template that I can use to generate an inital cobra application.
We will use the cookie template to generate depot
cli application to handle different types of storage like aws
, fs
, gcloud
, and azure
.
We won’t be implementing CRUD operations for each storage type. We will mock out functionality using print statements to demonstrate Cobra’s subcommand capabilities.
Steps Link to heading
My Cookiecutter blog post can help with setting up the inital cobra application
Once you have setup the cli application you can run these commands to generate subcommands e.g.
go run main.go aws
cobra-cli add aws cobra-cli add fs cobra-cli add azure cobra-cli add gcloud
Output if you run
go run main.go -h
➜ depot git:(main) ✗ go run main.go -h A longer description that spans multiple lines and likely contains examples and usage of using your application. For example: Cobra is a CLI library for Go that empowers applications. This application is a tool to generate the needed files to quickly create a Cobra application. Usage: depot [command] Available Commands: aws A brief description of your command azure A brief description of your command completion Generate the autocompletion script for the specified shell fs A brief description of your command gcloud A brief description of your command help Help about any command Flags: -h, --help help for depot -t, --toggle Help message for toggle Use "depot [command] --help" for more information about a command.
Add these commands into
crud.go
and make sure to register them e.g.func init() { rootCmd.AddCommand(awsCmd) awsCmd.AddCommand(CpCmd) awsCmd.AddCommand(DeleteCmd) awsCmd.AddCommand(ListCmd) }
Here is the output if you run
go run main.go aws -h
. You should see cp, ls, delete subcommands➜ depot git:(main) ✗ go run main.go aws -h A longer description that spans multiple lines and likely contains examples and usage of using your command. For example: Cobra is a CLI library for Go that empowers applications. This application is a tool to generate the needed files to quickly create a Cobra application. Usage: depot aws [flags] depot aws [command] Available Commands: cp A brief description of your command delete A brief description of your command ls A brief description of your command Flags: -h, --help help for aws Use "depot aws [command] --help" for more information about a command.
Execute sub-sub commands to verify functionality.
➜ depot git:(main) go run main.go aws ls cp called ➜ depot git:(main) ✗ go run main.go aws ls ls called ➜ depot git:(main) ✗ go run main.go aws delete delete called
Congrulations, you now have a cobra cli application with various subcommands where you can extend functionality if you would like
Learnings Link to heading
With Cobra, I feel it follows a Makefile approach where you can organized your tooling in way that makes logical sense to the user
APPNAME VERB NOUN --ADJECTIVE
In combination with the golang programming language where you can generate executables based on the hardware and os, this cli framework lend itself to generate powerful cli tools.