I am currently delving into Golang libraries, and I must say, the depth and breadth of resources available are impressive. Many of my needs can be met directly within these libraries. For instance, creating a basic server is straightforward, and the scalability options are robust. Today, I’m particularly intrigued by an external framework called Gin, which has garnered a lot of attention from developers. I’m eager to explore it firsthand to understand the rationale behind its creation and how it improves upon the native Golang net/http module.
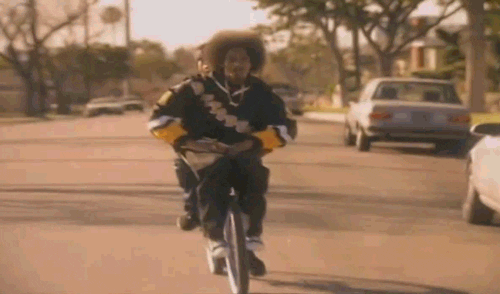
Using my cookiecutter template I can create a simple gin api
With my cookie-cutter template, I got a simple gin API that can respond to the GET HTTP endpoint call to get all messages and the POST-HTTP endpoint to create a new message.
GIN’s simplistic nature got my attention because GIN takes care of the serialization and deserialization. All you have to worry about is the business logic.
func handlerGetMessages(c *gin.Context) {
c.JSON(http.StatusOK, MessageResponse{
Messages: messages,
})
}
func handlerCreateMessage(c *gin.Context) {
var requestBody MessageRequest
if err := c.BindJSON(&requestBody); err != nil {
c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()})
return
}
if requestBody.Message == "" {
c.JSON(http.StatusBadRequest, gin.H{"error": "Empty message"})
}
messages = append(messages, requestBody.Message)
c.JSON(http.StatusCreated, gin.H{"message": "Message created successfully"})
}
Not much deviates from the http.Server
approach that comes out of the box from Golang. As of right now, all I have is a template to create
a simple gin API server. I will look into how to set up middleware like Prometheus, authentication, or logging. I will promote my cookie-cutter
templates with new Golang learnings as I go.