The problem I am trying to solve is that I was trying to find another way to use kustomize build
within my golang applications
One way to do it is install kustomize
and then leverage the os/exec
package to execute the kustomize CLI command from within your golang application. I feel this method is a bit hacky and less flexible in the way errors are handled.
Since kustomize is built using golang, why not used the native apis to run kustomize. Kustomize has this krusty API that you can create your own kustomizer. Using the native apis will give me better error handling and integration with leveraging the other cool features that golang has.
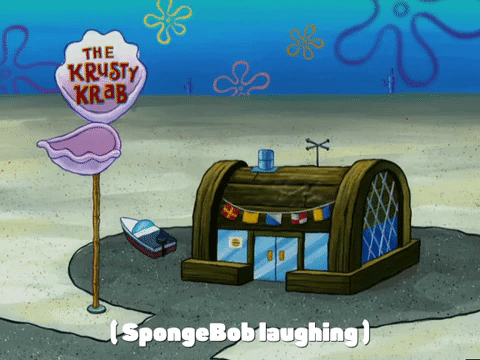
I have a Github repository that contains example of using this API. In this example. I do the following
- Look for folders that have an overlays folder
- Grab overlays’s subdirectories
- Validate these subdirectories’
kustomization.yaml
is valid
Here is a snippet of the code itself.
|
|
Line 19 - 30 showcase creating a kustomizer where you can read and validate kustomization.yaml
file
Nothing to fancy but you can get fancy like Flux Kustomize Controller with their own kubernetes operator listening for kustomize custom resource definitions
Learnings Link to heading
Using the krusty
api showed me that extending kustomize functionality is possible and beneficial for Platform teams who manage bulk kustomization files to add their own custom validation and manipulation logic to ensure certain file standards.